The Runge-Kutta Program
This program solves a system of differential equations. Higher order equations must be broken down into first-order equations before you can use the program.
Introduction
The Runge-Kutta program uses a fifth-order Runge-Kutta method to solve a system of first-order differential equations of the type y′ = f( x,y ). The program finds approximate solutions at particular points x0, x1, …, xn where the difference between successive x values is a constant, h = xn+1 - xn.You must enter the differential equations as subroutines labeled f1, f2, .. fn (a maximum of nine) before running the program. The value of the independent variable must be recalled from register E (004). The other variables correspond to registers L, M, …, T (011-019).
The program requires you to reduce higher-order differential equations with an extra variable from each order of derivative so that no variable is differentiated more than once.
For example, the third-order equation y′′′ = x + y is defined in terms of first-order equations as follows.
y = y1 | Substituting gives: |
y′ = y2 | y1′ = y2 |
y′′ = y3 | y2′ = y3 |
y′′′ = x + y1 | y3′= x + y1 |
You would describe these equations with the subroutines below.
LBL f1 | LBL f2 | LBL f3 |
RCL M | RCL N | ( RCL E + RCL L ) |
RTN | RTN | RTN |
For the simultaneous solution of several differential equations, you can substitute y1 … yn for the existing variables in first-order equations and reduce higher-order equations as shown previously. For example, the equations z′ = e-5x and y′′ = -y′ - 2.5y are written as follows.
z = y1 | Substituting gives: |
y = y2 | y1′ = e-5x |
y′ = y3 | y2′ = y3 |
y′′ = -y′ - 2.5y | y3′= -y3 + 2.5*y2 |
These equations written as subroutines are:
LBL f1 | LBL f2 | LBL f3 |
( 5 +/- * | RCL N | ( RCL N +/- |
RCL E ) INV LNX | RTN | - 2.5 * RCL M ) |
RTN | RTN |
You must partition the calculato memory for at least 83 data registers before running the program.
The program prompts you for the following parameters.
- The number of functions in the system of differential equations tells the program which subroutines to include when performing the solution.
- The h value (step size of x's) has an effect on the accuracy of the solution. A smaller h generally yields greater accuracy but slows the solution.
- The starting and ending x values define the boundaries for x.
- The initial value of each derivative provies boundary conditions necessary for the solution.
Reference
Numerical Solutions of Ordinary Differential Equations, Lapidus and Seinfeld.For the Beginning Programmer
Before running the Runge-Kutta program, you must store the functions in program memory as subroutines.If you are not familiar with keystroke programming, refer to the following chapters in the RPD-95 Programming Guide for instructions.
- "Working with Programs on the RPD-95"
- "Using Calculator Keystrokes in a Program"
- "Controlling the Sequence of Operations"
Rules for Storing the Functions
When you store the functions as a subroutines, follow these rules.- The subroutines must be labeled beginning with f1 (lowercase only) and continuing through the last function, up to a maximum of nine (9) functions.
- Anytime the subroutine needs the x variable, use a RCL
E or RCL 004 instruction. The program
stores the values of the dependent variables as follows:
Dependent Variable
Register
y1 L (011) y2 M (012) y3 N (013) y4 O (014) y5 P (015) y6 Q (016) y7 R (017) y8 S (018) y9 T (019) - Each subroutine must terminate with a RTN instruction.
Starting the Program
To start the Runge-Kutta program:- Store the functions needed by the program, following the rules given above.
- If any of the subroutines involve any of the trig functions, select the angle units you want to use.
- Select { R-K } from the
MATHEMATICS menu.
The program displays:
- Enter the number of functions stored and press { n }.
- Enter the step size and press { h }.
- Enter the initial value of x and press { LOx }.
- Enter the ending value of x and press { HIx }.
- Press { EOD }.
Entering the Initial Values
When you press { EOD }, the program prompts you to enter the initial value of y for each function.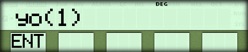
Enter all the initial values of y, pressing { ENT } after each value.
The Edit Menu
After you enter the initial values of y, the program displays an EDIT? menu that allows you to change any of the values.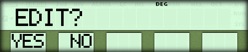
- If you do not want to edit, press { NO } and go the the section "Choosing the Results".
- If you want to edit, press { YES } and use the procedure listed in the next section below.
Editing the Initial Values
When you select { YES } from the EDIT? menu, the program displays the same menu that you used to enter the initial values.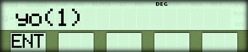
- To display the current value, press the [ CE ] key.
- To accept the current value and proceed to the next y value, press { ENT }.
- To edit the current value, enter the correct value and press { ENT }.
Choosing the Results
When you select { NO } from the EDIT? menu, the program displays: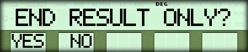
- To display the y values at each increment between the low and high values of x, press { NO } and go the section "Displaying Incremental Results".
- To display the y values at the high value of x only, press { YES } and use the procedure listed in the section "Displaying End Results Only" below.
Displaying End Results Only
When you press { YES }, the program displays: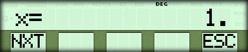
- Press { NXT }.
The program displays the corresponding y values, beginning with the y vaue for the first function.
for example.
- Press { NXT } repeatedly to display the y value for each remaining function.
Displaying Incremental Results
When you select { NO } from the END RESULT ONLY? menu, the program displays the first incremental value of x.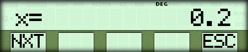
- Press { NXT }.
The program displays the corresponding y values, beginning with the y value for the first function.
for example.
- Press { NXT } repeatedly to display the y
value for each remaining function.
When the y value for the last function is displayed, pressing { NXT } redisplays the next incremental value of x. - Repeat steps 1 and 2 until all values of x and y have been displayed.
☚ Back