Using Subroutines in a Program
As you begin to write programs that are more complex, you may find that you need to execute the same sequence of instructions from several locations in the program. Although you could store the sequence at each point where you want it to execute, you can save yourself time by designing the sequence as a subroutine and storing it only once.
What Is A Subroutine?
A subroutine is a sequence of instructions that can be executed, or called, from any point in the program. When you call a subroutine, control is transferred to the beginning of the subroutine. After the subroutine has executed, control returns to the instruction that follows the subroutine call. The word "call" is understood by programmers to mean that control will return to the original segment after the subroutine has been executed.To include a subroutine in a program, use the following procedure.
- Store a label at the beginning of the subroutine. (Although you can transfer control to the step address of a subroutine, using a label has the advantages stated earlier.)
- Store the instructions that make up the subroutine.
- Store a RTN (return) instruction at the end of the subroutine. To enter a return instruction, press [ 2nd ] [ RTN ].
The following illustrations shows how a subroutine affects the flow of program execution. The SBL instruction (described later in this section) is used to call the subroutine.
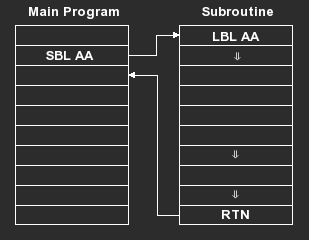
Levels of Subroutines
You can design a program so that one subroutine calls another subroutine. In this case, the RTN instruction at the end of the second subroutine returns control to the first subroutine, which returns control to the original program segment.The calculator allows a maximum of eight "levels" of subroutines to be active at one time. The illustration below shows two levels of subroutine calls.
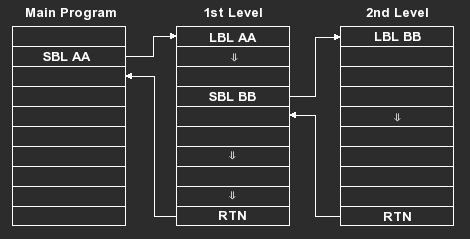
When the calculator encounters a RTN instruction, it returns control to the program segment or subroutine that called the current level of subroutine. If the calculator encounters a RTN instruction when no levels are active, the program stops. (RTN operates like HLT if there are no subroutine levels active.)
Calling a Subroutine by Label
The SBL (subroutine label) instruction transfers program control to a subroutine that begins with a specified label.To enter the SBL instruction, use the key sequence
[ 2nd ] [ SBL ] aa
where aa represents the label of the subroutine you want to call.
After the subroutine has executed, program control returns to the instruction following the SBL instruction.
Calling a Subroutine by Address
The SBR (subroutine) instruction lets you transfer program control to a subroutine by referring to the subroutine's program address.To enter the SBR instruction, use the key sequence
[ INV ] [ 2nd ] [ SBL ] nnnn
where nnnn represents the step address of the first instruction in the subroutine.
After the subroutine has executed, program control returns to the instruction following the SBR instruction.
Avoiding Difficulties in Subroutines
To avoid some common problems that can occur when using subroutines in programs, keep these suggestions in mind.- To prevent the accidental execution of a subroutine, make sure the program segment preceding it ends with a RTN, HLT, or transfer instruction.
- If the subroutine needs an intermediate result, use parentheses instead of [ = ] to perform the calculation. This avoids completing calculations in progress in the program segment that called the subroutine.
- If you need to clear the display within a subroutine, use a numeric entry of 0 instead of [ CLEAR ]. [ CLEAR ] clears all calculations in progress.
- You should not use a subroutine to call itself. Using a subroutine to call itself will generally result in a SBR STACK FULL error.
Example
This example illustrates a simple subroutine, labeled PZ, that multiplies the contents of the numeric display register by 2, adds 1, and pauses for one second before returning control to the main program. The main program calls the subroutine to perform the calculation on the numbers 2 and 9.PC = | Program Mnemonics | Comments |
---|---|---|
0000 | LBL AA | Labels segment |
0003 | CLR | Clears calculator |
0004 | 2 SBL PZ | Calls subroutine |
0008 | 9 SBL PZ | Calls subroutine again |
0012 | CLR | Clears calculator |
0013 | HLT | Stops program |
0014 | LBL PZ | Labels subroutine |
0017 | (*2+1) | Performs calculation |
0023 | PAU | Pauses to display result |
0024 | RTN | Returns from subroutine |
Running the Example
Run the program.Procedure | Press | Display |
---|---|---|
Run the program | [ RUN ] { PGM } | ![]() |
![]() | ||
![]() |
☚ Back