Example: Using CIO to Control a Peripheral
This section gives an example of using the CIO function to control the (PC-324) printer emulation. In actual use, you can control the printer directly from the keyboard; you are not required to use the CIO function. The program does, however, illustrate how to use the function.
Setting Up the Program
The sample program prints the message HELLO THERE five times, using the double-spacing option of the PC-324 printer emulation. It uses subroutines to send the necessary I/O commands - Open, Close, and Write - to the printer.The device number for the PC-324 printer emulation is usually given as the decimal number 12. To enter this value into a PAB, the program uses the hexadecimal equivalent, which is 0C.
Before writing such a program, you must determine which registers you want to use for the PAB, the data buffer, and any variables needed by the program. (Because this program uses a loop, it needs one register for the loop counter.)
- Reg A (000) - Loop Counter
- Reg B (001) - PAB (bytes 1 through 8)
- Reg C (002) - PAB (bytes 9 through 12)
- Reg D (003) - Beginning of the data buffer
The PAB requires the hexadecimal address of the first byte in the data buffer. By using the formula in Appendix A, you can calculate that the first byte in data register 003 is at address 3FE7 (this information is directly obtainable from the Register View).
The example uses the following labels for the subroutines.
- LBL OP - Subroutine for OPEN command
- LBL CL - Subroutine for CLOSE command
- LBL WR - Subroutine for WRITE command
The Main Program
The main program segment calls the three subroutines listed on the next few pages. This segment sets the halt-on-error flag because the program does not do its own error checking.Note:
This program begins by closing the printer. This is required because the RPD-95 automatically opens the PC-324 printer emulation if the printer is connected (enabled) when you turn on the calculator. Because the program closes the printer at both the beginning and the end, attempting to run the program a second time produces I/O error 4 (not open). Before the program can be run a second time, the calculator must be turned off and then on.Program Mnemonics | Comments |
---|---|
SF 15 | Sets halt-on-error flag |
SBL CL | Close printer |
SBL OP | Open printer |
`HELLO THERE` | Alpha message |
STA D | Store message in data buffer |
5 STO A | Set loop counter to 5 |
LBL AA | |
SBL WR | Write message |
DSZ A | |
GTL AA | |
SBL CL | Close printer at end of loop |
HLT | Halt program |
Selecting Options for the Open Command
Before you send an Open command, you need to know the following information in order to store it in the data buffer.- Requested I/O buffer size - The PC-324 printer emulation uses a default buffer size of 24 bytes, which is equivalent to the two byte hex value 0018. (In a case where you prefer to let a device establish the maximum buffer size for you, use 0000 in this field, and read the returned value after the Open command has successfully executed.)
- Device Attributes - Use the OUTPUT access mode and, because the printer does not support files, use zeros for the remaining bits. The resulting byte value is 80 hex.
- Device Options - The double-spacing option is specified by the character string "L=D". The ASCII values for these three characters are 4C3D44.
For the Open command in this example, the data buffer should contain the following values.
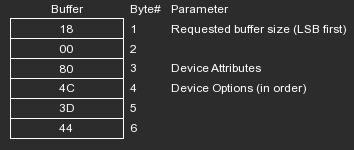
Subroutine for the Open Command
For the Open command, the PAB contains the values shown below.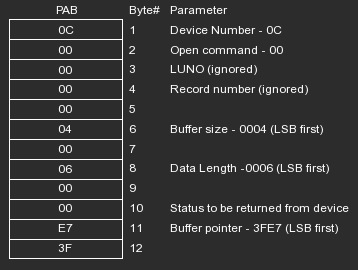
The following subroutine loads the data buffer, builds the PAB, and sends the Open command.
Program Mnemonics | Comments |
---|---|
LBL OP | |
UNF | Selects unformatted mode |
1800804C3D44 | Contents of data buffer |
STO D | |
0C00000000040006 | First 8 bytes of PAB |
STO B | |
0000E73F | Remaining 4 bytes of PAB |
STO C | |
DEC | Restores decimal mode |
CIO B | Sends the command |
RTN |
Subroutine for the Close Command
For the Close command, the PAB contains the values shown below.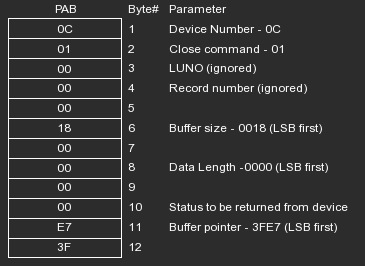
The following subroutine builds this PAB and sends the Close command.
Program Mnemonics | Comments |
---|---|
LBL CL | |
UNF | Selects unformatted mode |
0C01000000000018 | First 8 bytes of PAB |
STO B | |
0000E73F | Remaining 4 bytes of PAB |
STO C | |
DEC | Restores decimal mode |
CIO B | Sends the command |
RTN |
Subroutine for the Write Command
For the Write Command, the PAB contains the values shown below.Because the data consists of the 11 characters HELLO THERE, the program specifies 0B (the hex equivalent of 11) as the data length.
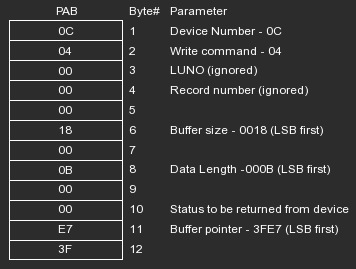
The following subroutine builds this PAB and sends the Write command. (The main program has already stored the message in the data buffer.)
Program Mnemonics | Comments |
---|---|
LBL WR | |
UNF | Selects unformatted mode |
0C0400000018000B | First 8 bytes of PAB |
STO B | |
0000E73F | Remaining 4 bytes of PAB |
STO C | |
DEC | Restores decimal mode |
CIO B | Sends the command |
RTN |
☚ Back